Note
Click here to download the full example code
Bilinear InterpolationΒΆ
This example shows how to use the pylops.signalprocessing.Bilinar
operator to perform bilinear interpolation to a 2-dimensional input vector.
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.gridspec as pltgs
from scipy import misc
import pylops
plt.close('all')
np.random.seed(0)
First of all, we create a 2-dimensional input vector containing an image
from the scipy.misc
family.
We can now define a set of available samples in the first and second direction of the array and apply bilinear interpolation.
At this point we try to reconstruct the input signal imposing a smooth solution by means of a regularization term that minimizes the Laplacian of the solution.
D2op = pylops.Laplacian((nz, nx), weights=(1, 1), dtype='float64')
xadj = Bop.H * y
xinv = pylops.optimization.leastsquares.NormalEquationsInversion(Bop, [D2op], y,
epsRs=[np.sqrt(0.1)],
returninfo=False,
**dict(maxiter=100))
xadj = xadj.reshape(nz, nx)
xinv = xinv.reshape(nz, nx)
fig, axs = plt.subplots(1, 3, figsize=(10, 4))
fig.suptitle('Bilinear interpolation', fontsize=14,
fontweight='bold', y=0.95)
axs[0].imshow(x, cmap='gray_r', vmin=0, vmax=250)
axs[0].axis('tight')
axs[0].set_title('Original')
axs[1].imshow(xadj, cmap='gray_r', vmin=0, vmax=250)
axs[1].axis('tight')
axs[1].set_title('Sampled')
axs[2].imshow(xinv, cmap='gray_r', vmin=0, vmax=250)
axs[2].axis('tight')
axs[2].set_title('2D Regularization')
plt.tight_layout()
plt.subplots_adjust(top=0.8)
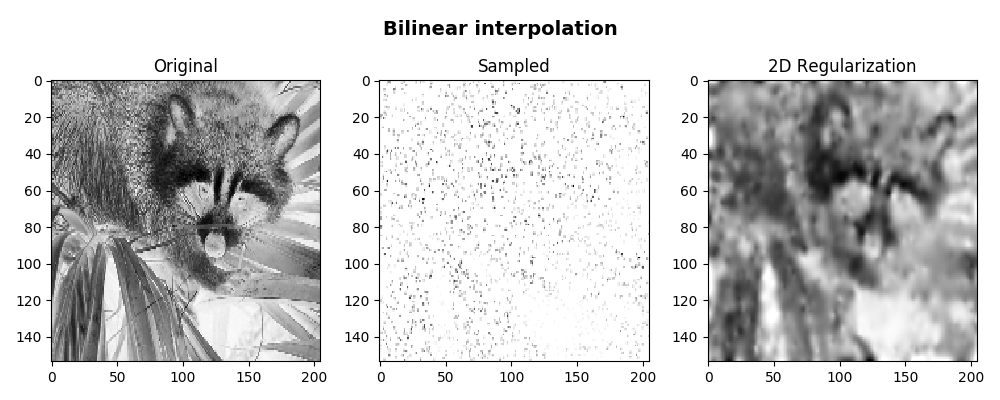
Out:
/home/docs/checkouts/readthedocs.org/user_builds/pylops/envs/v1.8.0/lib/python3.6/site-packages/pylops-1.8.0-py3.6.egg/pylops/signalprocessing/Bilinear.py:117: FutureWarning: Using a non-tuple sequence for multidimensional indexing is deprecated; use `arr[tuple(seq)]` instead of `arr[seq]`. In the future this will be interpreted as an array index, `arr[np.array(seq)]`, which will result either in an error or a different result.
/home/docs/checkouts/readthedocs.org/user_builds/pylops/envs/v1.8.0/lib/python3.6/site-packages/pylops-1.8.0-py3.6.egg/pylops/signalprocessing/Bilinear.py:119: FutureWarning: Using a non-tuple sequence for multidimensional indexing is deprecated; use `arr[tuple(seq)]` instead of `arr[seq]`. In the future this will be interpreted as an array index, `arr[np.array(seq)]`, which will result either in an error or a different result.
/home/docs/checkouts/readthedocs.org/user_builds/pylops/envs/v1.8.0/lib/python3.6/site-packages/pylops-1.8.0-py3.6.egg/pylops/signalprocessing/Bilinear.py:121: FutureWarning: Using a non-tuple sequence for multidimensional indexing is deprecated; use `arr[tuple(seq)]` instead of `arr[seq]`. In the future this will be interpreted as an array index, `arr[np.array(seq)]`, which will result either in an error or a different result.
/home/docs/checkouts/readthedocs.org/user_builds/pylops/envs/v1.8.0/lib/python3.6/site-packages/pylops-1.8.0-py3.6.egg/pylops/signalprocessing/Bilinear.py:123: FutureWarning: Using a non-tuple sequence for multidimensional indexing is deprecated; use `arr[tuple(seq)]` instead of `arr[seq]`. In the future this will be interpreted as an array index, `arr[np.array(seq)]`, which will result either in an error or a different result.
Total running time of the script: ( 0 minutes 3.476 seconds)